- xは
行の真偽値表(2列)
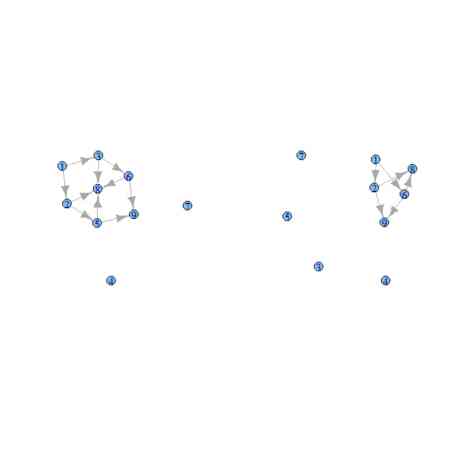
library(igraph)
bzdd <- function(x,type="zdd"){
v <- c(x)
K <- log(length(v),2)
lay.y <- c()
for(i in 1:K){
lay.y <- c(lay.y,rep(i,2^(i-1)))
}
lay.y <- c(lay.y,rep(K+1,2))
g.tree <- graph.tree(sum(2^(0:(K-1))))
m.a <- as.matrix(get.adjacency(g.tree))
for(i in 1:length(m.a[,1])){
m.a[i,which(m.a[i,]==1)[2]] <- 2
}
m.a.2 <- matrix(0,length(m.a[,1])+2,length(m.a[1,])+2)
m.a.2[1:length(m.a[,1]),1:length(m.a[1,])] <- m.a
tmp.m <- matrix(v,ncol=2)
m.a.2[sum(2^(0:(K-2)))+which(tmp.m[,1]==0),length(m.a[1,])+1] <- 1
m.a.2[sum(2^(0:(K-2)))+which(tmp.m[,2]==0),length(m.a[1,])+1] <- m.a.2[sum(2^(0:(K-2)))+which(tmp.m[,2]==0),length(m.a[1,])+1] + 2
m.a.2[sum(2^(0:(K-2)))+which(tmp.m[,1]==1),length(m.a[1,])+2] <- 1
m.a.2[sum(2^(0:(K-2)))+which(tmp.m[,2]==1),length(m.a[1,])+2] <- m.a.2[sum(2^(0:(K-2)))+which(tmp.m[,2]==1),length(m.a[1,])+2] + 2
if(type=="zdd"){
m.a.zdd <- m.a.2
m.a.2 <- NULL
for(i in (K-1):0){
if(i==0){
ns <- c(1)
}else{
ns <- (sum(2^(0:(i-1))) + 1):sum(2^(0:i))
}
for(j in 1:length(ns)){
current <- ns[j]
tozero <- m.a.zdd[current,length(m.a.zdd[,1])-1]
if(tozero == 2 | tozero == 3){
for(jjj in 1:3){
coming <- which(m.a.zdd[,current]==jjj)
togo <- which(m.a.zdd[current,] == 1 | m.a.zdd[current,] == 3)
if(length(coming)>0){
m.a.zdd[coming,togo] <- m.a.zdd[coming,togo] + jjj
m.a.zdd[coming,current] <- 0
m.a.zdd[current,] <- 0
}
if(current == 1){
m.a.zdd[current,]<- 0
}
}
}
}
}
}else if(type=="bdd"){
for(i in (K-1):1){
ns <- (sum(2^(0:(i-1))) + 1):sum(2^(0:i))
for(j in 1:length(ns)){
current <- ns[j]
if(length(which(m.a.2[current,]>0))==1){
togo <- which(m.a.2[current,]>0)
for(jj in 1:3){
coming <- which(m.a.2[,current]==jj)
m.a.2[coming,togo] <- m.a.2[coming,togo] + jj
m.a.2[coming,current] <- 0
m.a.2[current,] <- 0
}
}else if(length(which(m.a.2[current,]>1))==2){
if(j < length(ns)-1){
for(jj in (j+1):length(ns)){
if(sum(abs(m.a.2[current,]-m.a.2[ns[jj],]))==0){
togo <- ns[jj]
for(jjj in 1:3){
coming <- which(m.a.2[,current]==jjj)
m.a.2[coming,togo] <- m.a.2[coming,togo] + jjj
m.a.2[coming,current] <- 0
m.a.2[current,] <- 0
}
}
}
}
}
}
}
}else if(type == "both"){
m.a.zdd <- m.a.2
for(i in (K-1):0){
if(i==0){
ns <- c(1)
}else{
ns <- (sum(2^(0:(i-1))) + 1):sum(2^(0:i))
}
for(j in 1:length(ns)){
current <- ns[j]
if(length(ns)>1){
if(length(which(m.a.2[current,]>0))==1){
togo <- which(m.a.2[current,]>0)
for(jj in 1:3){
coming <- which(m.a.2[,current]==jj)
m.a.2[coming,togo] <- m.a.2[coming,togo] + jj
m.a.2[coming,current] <- 0
m.a.2[current,] <- 0
}
}else if(length(which(m.a.2[current,]>1))==2){
if(j < length(ns)-1){
for(jj in (j+1):length(ns)){
if(sum(abs(m.a.2[current,]-m.a.2[ns[jj],]))==0){
togo <- ns[jj]
for(jjj in 1:3){
coming <- which(m.a.2[,current]==jjj)
m.a.2[coming,togo] <- m.a.2[coming,togo] + jjj
m.a.2[coming,current] <- 0
m.a.2[current,] <- 0
}
}
}
}
}
}
tozero <- m.a.zdd[current,length(m.a.zdd[,1])-1]
if(tozero == 2 | tozero == 3){
for(jjj in 1:3){
coming <- which(m.a.zdd[,current]==jjj)
togo <- which(m.a.zdd[current,] == 1 | m.a.zdd[current,] == 3)
if(length(coming)>0){
m.a.zdd[coming,togo] <- m.a.zdd[coming,togo] + jjj
m.a.zdd[coming,current] <- 0
m.a.zdd[current,] <- 0
}
if(current == 1){
m.a.zdd[current,]<- 0
}
}
}
}
}
}
return(list(bdd.m = m.a.2,zdd.m = m.a.zdd))
}
x <- matrix(c(0,0,1,0,0,1,0,0),byrow=TRUE,ncol=2)
bzdd.out <- bzdd(x,type="both")
make.complex <- function(x){
n <- length(x[,1])
if(n==1){
return(NULL)
}
m <- matrix(0,n,n)
selected <- rep(1,n)
for(i in 1:n){
tmp <- apply(x,1,"-",x[i,])
same <- apply((tmp==0),2,prod)
bigger <- apply((tmp<=0),2,prod)
smaller <- apply((tmp>=0),2,prod)
if(length(which((smaller==1) & !(same==1)))>0){
selected[i] <- 0
}
if(length(which(same[1:i]==1))>1){
selected[i] <-0
}
m[i,which(bigger==1)] <- 2
m[i,which(smaller==1)] <- 3
m[i,which(same==1)] <- 1
}
tmp <- which(selected==1)
if(length(tmp)==1){
x <- matrix(x[which(selected==1),],nrow=1)
}else{
x <- x[which(selected==1),]
}
return(x)
}
n <- 8
Omega <- 1:n
k<- 5
x <- matrix(0,k,n)
for(i in 1:k){
x[i,sample(Omega,sample(1:(n/2),1))] <- 1
}
x.c <- make.complex(x)
b <- as.matrix(expand.grid(rep(list(0:1),n)))
b <- b[,n:1]
x.c <- x.c[,n:1]
vv <- rep(0,length(b[,1]))
for(i in 1:length(b[,1])){
tmp <- t(x.c) - b[i,]
judge <- FALSE
for(j in 1:length(tmp[1,])){
if(length(which(tmp[,j]==-1))==0){
judge <- TRUE
}
}
if(judge){
vv[i] <- 1
}
}
x <- matrix(c(0,0,1,0,0,1,0,0),byrow=TRUE,ncol=2)
bzdd.out <- bzdd(x,type="both")
bdd <- graph.adjacency(sign(bzdd.out$bdd.m))
zdd <- graph.adjacency(sign(bzdd.out$zdd.m))
par(mfcol=c(1,2))
plot(bdd)
plot(zdd)
par(mfcol=c(1,1))